Computing Higher Order Derivatives
To compute higher derivatives of a function f, we use the syntax diff(f,n).
Let us compute the second derivative of the function y = f(x) = x .e-3x
f = x*exp(-3*x); diff(f, 2)
MATLAB executes the code and returns the following result −
ans = 9*x*exp(-3*x) - 6*exp(-3*x)
Following is Octave equivalent of the above calculation −
pkg load symbolic symbols x = sym("x"); f = x*Exp(-3*x); differentiate(f, x, 2)
Octave executes the code and returns the following result −
ans = (9.0)*exp(-(3.0)*x)*x-(6.0)*exp(-(3.0)*x)
Example
In this example, let us solve a problem. Given that a function y = f(x) = 3 sin(x) + 7 cos(5x). We will have to find out whether the equation f" + f = -5cos(2x) holds true.
Create a script file and type the following code into it −
syms x y = 3*sin(x)+7*cos(5*x); % defining the function lhs = diff(y,2)+y; %evaluting the lhs of the equation rhs = -5*cos(2*x); %rhs of the equation if(isequal(lhs,rhs)) disp('Yes, the equation holds true'); else disp('No, the equation does not hold true'); end disp('Value of LHS is: '), disp(lhs);
When you run the file, it displays the following result −
No, the equation does not hold true Value of LHS is: -168*cos(5*x)
Following is Octave equivalent of the above calculation −
pkg load symbolic symbols x = sym("x"); y = 3*Sin(x)+7*Cos(5*x); % defining the function lhs = differentiate(y, x, 2) + y; %evaluting the lhs of the equation rhs = -5*Cos(2*x); %rhs of the equation if(lhs == rhs) disp('Yes, the equation holds true'); else disp('No, the equation does not hold true'); end disp('Value of LHS is: '), disp(lhs);
Octave executes the code and returns the following result −
No, the equation does not hold true Value of LHS is: -(168.0)*cos((5.0)*x)
Finding the Maxima and Minima of a Curve
If we are searching for the local maxima and minima for a graph, we are basically looking for the highest or lowest points on the graph of the function at a particular locality, or for a particular range of values of the symbolic variable.
For a function y = f(x) the points on the graph where the graph has zero slope are called stationary points. In other words stationary points are where f'(x) = 0.
To find the stationary points of a function we differentiate, we need to set the derivative equal to zero and solve the equation.
Example
Let us find the stationary points of the function f(x) = 2x3 + 3x2 − 12x + 17
Take the following steps −
First let us enter the function and plot its graph.
syms x y = 2*x^3 + 3*x^2 - 12*x + 17; % defining the function ezplot(y)
MATLAB executes the code and returns the following plot −
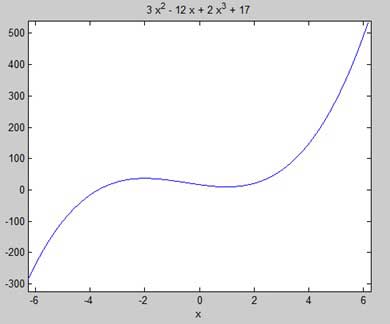
Here is Octave equivalent code for the above example −
pkg load symbolic symbols x = sym('x'); y = inline("2*x^3 + 3*x^2 - 12*x + 17"); ezplot(y) print -deps graph.eps
Our aim is to find some local maxima and minima on the graph, so let us find the local maxima and minima for the interval [-2, 2] on the graph.
syms x y = 2*x^3 + 3*x^2 - 12*x + 17; % defining the function ezplot(y, [-2, 2])
MATLAB executes the code and returns the following plot −
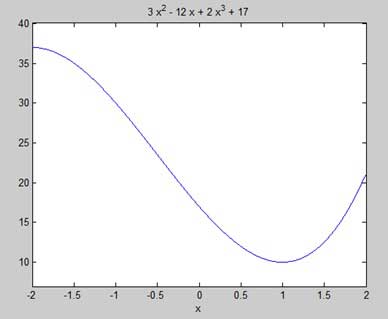
Here is Octave equivalent code for the above example −
pkg load symbolic symbols x = sym('x'); y = inline("2*x^3 + 3*x^2 - 12*x + 17"); ezplot(y, [-2, 2]) print -deps graph.eps
Next, let us compute the derivative.
g = diff(y)
MATLAB executes the code and returns the following result −
g = 6*x^2 + 6*x - 12
Here is Octave equivalent of the above calculation −
pkg load symbolic symbols x = sym("x"); y = 2*x^3 + 3*x^2 - 12*x + 17; g = differentiate(y,x)
Octave executes the code and returns the following result −
g = -12.0+(6.0)*x+(6.0)*x^(2.0)
Let us solve the derivative function, g, to get the values where it becomes zero.
s = solve(g)
MATLAB executes the code and returns the following result −
s = 1 -2
Following is Octave equivalent of the above calculation −
pkg load symbolic symbols x = sym("x"); y = 2*x^3 + 3*x^2 - 12*x + 17; g = differentiate(y,x) roots([6, 6, -12])
Octave executes the code and returns the following result −
g = -12.0+(6.0)*x^(2.0)+(6.0)*x ans = -2 1
This agrees with our plot. So let us evaluate the function f at the critical points x = 1, -2. We can substitute a value in a symbolic function by using the subs command.
subs(y, 1), subs(y, -2)
MATLAB executes the code and returns the following result −
ans = 10 ans = 37
Following is Octave equivalent of the above calculation −
pkg load symbolic symbols x = sym("x"); y = 2*x^3 + 3*x^2 - 12*x + 17; g = differentiate(y,x) roots([6, 6, -12]) subs(y, x, 1), subs(y, x, -2)
ans = 10.0 ans = 37.0-4.6734207789940138748E-18*I
Therefore, The minimum and maximum values on the function f(x) = 2x3 + 3x2− 12x + 17, in the interval [-2,2] are 10 and 37.
Solving Differential Equations
MATLAB provides the dsolve command for solving differential equations symbolically.
The most basic form of the dsolve command for finding the solution to a single equation is
dsolve('eqn')
where eqn is a text string used to enter the equation.
It returns a symbolic solution with a set of arbitrary constants that MATLAB labels C1, C2, and so on.
You can also specify initial and boundary conditions for the problem, as comma-delimited list following the equation as −
dsolve('eqn','cond1', 'cond2',…)
For the purpose of using dsolve command, derivatives are indicated with a D. For example, an equation like f'(t) = -2*f + cost(t) is entered as −
'Df = -2*f + cos(t)'
Higher derivatives are indicated by following D by the order of the derivative.
For example the equation f"(x) + 2f'(x) = 5sin3x should be entered as −
'D2y + 2Dy = 5*sin(3*x)'
Let us take up a simple example of a first order differential equation: y' = 5y.
s = dsolve('Dy = 5*y')
MATLAB executes the code and returns the following result −
s = C2*exp(5*t)
Let us take up another example of a second order differential equation as: y" - y = 0, y(0) = -1, y'(0) = 2.
dsolve('D2y - y = 0','y(0) = -1','Dy(0) = 2')
MATLAB executes the code and returns the following result −
ans = exp(t)/2 - (3*exp(-t))/2